How Do You Know Dups Values in the List Interface
JavaScript gives developers a great deal of flexibility. A variable initialized every bit an integer can be assigned a function literal at run-time. Types of variables are not predictable in JavaScript. As you tin come across in the case below, a
is initialized as an integer and is then assigned a function literal:
var a = 2 a = function () { panel.log("I was initialized with a value of an integer, but now I'yard a part") }
Allow's consider the implementation plan for building a Tesla Model S car.
Ten of the Tesla engineers built their paradigm model. There were no specifications laid down before implementation, and then the engineers all came up with their own ready of specifications and implementation model. One of these prototypes had the means to testify a user the automobile charging details while the other i had a tire monitoring system in place.
If a set of specifications were divers beforehand, it would have been convenient and piece of cake for these engineers to implement prototypes based on the specifications. We deal with the same problem while building complex entities in JavaScript:
function buildTeslaModelS (teslaObj) { // Implementation Details } buildTeslaModelS({ length: 196, width: 86, measureTirePressure: function () { }, wheelbase: 116 }) buildTeslaModelS({ length: "196", width: "86", wheelbase: "116", measureRemCharging: function () { } })
The buildTeslaModelS
function returns a Tesla Model Southward car using the parameters equally defined in teslaObj
. It makes some assumptions for input parameters and returns a model based on those assumptions. It assumes that the length
, width
, and wheelbase
properties would be integers, and it performs some computations based on this assumption.
However, as you can come across in the second function phone call to buildTeslaModelS
, these values are strings, and then the assumption is no longer valid.
Also, the buildTeslaModelS
role doesn't know that it would take to bargain with the measureRemCharging
holding, and then information technology completely skips that part. It assumes that measureTirePressure
is a mandatory property and that information technology should be present in all of these models. However, when information technology doesn't find this property in the 2d function call, it throws an error at runtime.
This is an extremely flexible functionality! There should be a way to tell the buildTeslaModelS
part the shape of the input teslaObj
parameter. It would have been easier if there was a validation bank check for checking mandatory properties and their types on teslaObj
at compile time.
Hither come TypeScript interfaces to aid!
TypeScript has built-in support for interfaces. An interface defines the specifications of an entity. It lays out the contract that states what needs to be done simply doesn't specify how information technology volition be done.
In the higher up example, we can define an interface for the Tesla Model S, and each of its prototypes would so use this interface to come up with their implementation plan for various functionalities as defined in the interface.
This is the interface for the Tesla Model S:
interface TeslaModelS { length: number; width: number; wheelbase: number; seatingCapacity: number; getTyrePressure: () => number; getRemCharging: () => number; }
An interface contains the name of all the properties along with their types. It also includes the signature for functions forth with the type of arguments and return type. For instance, getTyrePressure
and getRemCharging
functions return the value of type number
.
How to apply an interface
A form or function can implement an interface to define the implementation of the properties as divers in that interface.
Let's write a function to implement TeslaModelS
interface:
function buildTeslaModelS (teslaObj: TeslaModelS) { } buildTeslaModelS({ length: "196", width: "86", wheelbase: "116", measureRemCharging: function () { } })
When you lot run the code shown above, the TypeScript compiler volition requite the following error:
Statement of type
{ length: string; width: string; wheelbase: cord; measureRemCharging: () => void; }
is non assignable to parameter of blazonTeslaModelS
. Object literal may just specify known backdrop, andmeasureRemCharging
does not exist in blazonTeslaModelS
.
The compiler complains for two reasons:
- The properties
length
,width
, andwheelbase
are divers as blazonnumber
in the interface, so it expects them to be of typenumber
and notstring
- The property
measureRemCharging
is not defined on the interface. It should be named asgetRemCharging
and it should return aninteger
. The implementation of an entity should follow the contract as defined in its interface
To build a Tesla Model South as defined in the interface, we will accept to define the function similar this:
function buildTeslaModelS (teslaObj: TeslaModelS) { } buildTeslaModelS({ length: 196, width: 86, wheelbase: 116, seatingCapacity: 4, getTyrePressure: office () { let tyrePressure = 20 // Evaluated later doing a few complex computations! render tyrePressure }, getRemCharging: function () { let remCharging = 20 // Evaluated later doing a few complex computations! return remCharging } })
The above implementation of teslaObj
is exactly what the interface expects!
How to define optional properties in interfaces
Interfaces do a great task in making sure the entities are implemented equally expected. However, there would be cases when it is not necessary to have all of the properties as defined in the interface. These are called optional properties and are represented in the interface like this:
interface TeslaModelS { length: number; width: number; wheelbase: number; seatingCapacity: number; getTyrePressure?: () => number; getRemCharging: () => number; }
Please annotation the?
in the getTyrePressure
property. The question marking suggests that the property getTyrePressure
is optional and is not mandatory for entities to implement this functionality in all models. The compiler won't complain fifty-fifty if you don't specify this property in the teslaObj
parameter.
The compiler also checks for excess properties that are not defined in the interface. Allow'due south say, the teslaObj
contains an backlog property turningCircle
, which is not specified in the TeslaModelS
interface:
buildTeslaModelS({ length: 196, width: 86, wheelbase: 116, getTyrePressure: function () { let tyrePressure = 20 // Evaluated after doing a few circuitous computations! return tyrePressure }, getRemCharging: office () { permit remCharging = 20 // Evaluated later doing a few complex computations! return remCharging }, turningCircle: x })
The compiler gives the post-obit error:
Argument of type
{ length: number; width: number; wheelbase: number; getTyrePressure: () => number; getRemCharging: () => number; turningCircle: number; }
is not assignable to parameter of blazonTeslaModelS
. Object literal may merely specify known properties, andturningCircle
does not be in typeTeslaModelS
.
Read-only backdrop in interfaces
Read-only properties cannot be changed once they are initialized. For instance, the properties length
, width
, wheelbase
, and seatingCapacity
should never be modified in any case after they are initialized with some stock-still value.
We will have to change our interface to reverberate this modify:
interface TeslaModelS { readonly length: number; readonly width: number; readonly wheelbase: number; readonly seatingCapacity: number; getTyrePressure?: () => number; getRemCharging: () => number; }
Note the use of the readonly
keyword with the proper name of the properties. It suggests that these properties cannot be modified after they are initialized with some value.
Indexable properties in interfaces
Indexable properties, as the name suggests are used for defining types that are indexed into a unique number or a string. For example, nosotros can define a type CustomArray
equally:
Please note the cars
variable is non an ordinary array so yous cannot apply array built-in functions like button
, pop
, filter
, etc. You might debate that information technology is better to define ordinary arrays instead of using indexable types. Indexable types are helpful when you have to define custom properties and functions that should operate on a range of values of the aforementioned data type.
Since we have clearly put together the specifications of the Tesla Model Southward in an interface, information technology has improved the efficiency of the Tesla engineers, and they are now set with the first set up of 100 cars. It is time for the reviewing committee to go through each of the models and exam them for performance and other factors:
The TeslaModelSReview
interface indexes the group of backdrop —engineer
, model
, and rating
associated with a item model into a unique numeric alphabetize. The TeslaModelSReviewQueue
is of blazon TeslaModelSReview
. It lists downward the Tesla models built by different engineers. From the above code, we can see that John has congenital two models —modelByJohn1
and modelByJohn2
, which are rated as 2
and 4
, respectively.
The type of indexer can either be a string or a number. We tin can besides define other backdrop in TeslaModelSReview
interface but these properties should return a subtype of TeslaModelS
type.
The indices of TeslaModelSReview
tin can be made read-but to prevent modifying its values while it is in the review process. We'll have to change our TeslaModelSReview
interface like this:
interface TeslaModelSReview { readonly [id: number]: TeslaModelS }
How to define function types in interfaces
An interface tin can as well be used for defining the structure of a role. Equally we saw earlier, the functions getTyrePressure
and getRemCharging
are defined equally properties on the TeslaModelS
interface. However, we tin can ascertain an interface for functions like this:
interface Order { (customerId: number, modelId: number): boolean } allow orderFn: Club = function (cId, mId) { // processing the club return true // processed successfully! }
The orderFn
role is to blazon Club
. It takes 2 parameters of type number
and returns a value of blazon boolean
.
There is no need to define the type of parameters over again in the definition of orderFn
function as yous tin can meet in the lawmaking above. The compiler just makes one-to-one mapping of the arguments as defined in the interface with the one divers in the part announcement.
Information technology infers that cId
maps to customerId
and its type is number
and mId
maps to modelId
and its type is as well number
. Fifty-fifty the return type for orderFn
function is inferred from its definition in the interface.
How to employ interfaces with classes
So far, we've learned how a function implements an interface. Now let's build a course for the TeslaModelS
interface.
class TeslaModelSPrototype implements TeslaModelS { length: number; width: number; wheelbase: number; seatingCapacity: number; private tempCache: string; constructor (l, w, wb, sc) { this.length = fifty; this.width = due west; this.wheelbase = wb; this.seatingCapacity = sc; } getTyrePressure () { allow tyrePressure = 20 // Evaluated after doing a few complex computations! return tyrePressure } getRemCharging () { let remCharging = xx // Evaluated after doing a few complex computations! return remCharging } } permit teslaObj = new TeslaModelSPrototype(196, 86, 116, four) console.log('Tyre Pressure', teslaObj.getTyrePressure())
The class TeslaModelSPrototype
has defined all the properties of an interface. Delight note, the interface defines simply the public backdrop of a course. As can exist seen from the above code, the property tempCache
has an access modifier private
so it is not defined in the interface TeslaModelS
.
Different types of variables in a class
A class has iii different types of variables
- Local variables: A local variable is divers at the part or block level. Information technology exists only until the function or the block is in execution. Every time a function runs, new copies of the local variables are created in memory
- Instance variables: Instances variables are members of the class. They are used to store the attributes of class objects. Each of the objects has its own copy of instance variables
- Static variables: Static variables are also called class variables because they are associated with a course equally a whole. All of the objects of a class share the same copy of static variables
Please note interfaces deal but with the instance role of the class. For example, the constructor
function comes nether the static
part. The interface TeslaModelS
does not specify anything related to the constructor
or the static role.
Extending interfaces
An interface tin can extend any other interface and import its properties. This helps in building small-scale and reusable components. For example, nosotros tin can create different interfaces to handle the unlike components of the Tesla Model like this:
interface Wheel { wheelBase: number; controls: Array<string>, material: cord; } interface Charger { adapter: string; connector: string; location: cord; } interface TeslaModelS extends Wheel, Charger { // ... All other properties }
The TeslaModelS
interface extends the properties of the Bike
and the Charger
. Instead of dumping all of the backdrop in a single interface, information technology is a proficient practise to make separate interfaces for handling different components.
How are type aliases different from interfaces?
Blazon aliases are used for giving a name to a combination of different types in TypeScript.
For instance, we can create a blazon that can either be of type string
or null
:
type StringOrNull = string | nix;
Type aliases and interfaces are frequently used interchangeably in TypeScript. The shape of the TeslaModelS
object can too exist defined using type
like this:
type TeslaModelS { length: number; width: number; wheelbase: number; seatingCapacity: number; getTyrePressure: () => number; getRemCharging: () => number; }
Similar to how interfaces extend other interfaces and type aliases using the keyword, type aliases can also extend other types and interfaces using the intersection operator. Type aliases can too be implemented by a form.
Blazon aliases are more often than not used in cases where we take to define a merge of dissimilar types. For example, consider the function renderObject
:
function renderObject (objShape: Square | Rectangle | Triangle) {\ // ... }
The
renderObject function takes an input parameter objShape
. Square
, Rectangle
, and Triangle
are types, and |
is called the spousal relationship operator. objShape
can be of type Square
, Rectangle
, or Triangle
. All the same, the marriage of shapes cannot be expressed using an interface.
Interfaces are used for defining a contract regarding the shape of an object; hence they cannot be used with the union of multiple shapes. Even a class cannot implement a type that describes a wedlock of shapes. This is one of the important functional differences betwixt interfaces and blazon allonym.
When nosotros define two interfaces with the same name, both of them gets merged into one. The resulting interface will accept properties from both the interfaces. However, the compiler will complain if nosotros try to define multiple types with the same name.
Hybrid types in interfaces
In JavaScript, functions are also considered equally objects and then it is valid to add backdrop even on function literals like this:
function manufactureCar (type) { const model = function getModel (blazon) { console.log('inside getModel function') // get the model of blazon equally mentioned in the argument } model.getCustomerDetails = function () { console.log('within client details role') // become the details of customer who has purchased this model } model.price = 100000 model.trackDelivery = function () { console.log('inside trackDelivery part') // track the delivery of the model } return model } allow tesla = manufactureCar('tesla') tesla() // tesla is a function tesla.getCustomerDetails() // getCustomerDetails is a property defined on function
Every bit yous tin can see from the above code, the variable model
is assigned a value of function and getCustomerDetails
, trackDelivery
are attached as properties on the model
. This is a common pattern in JavaScript. How practice we define this design with TypeScript interfaces?
interface CarDelivery { (string): TeslaModelS, getCustomerDetails (): cord, price: number, trackDelivery (): cord } part manufactureCar (type: string): CarDelivery { const model = <CarDelivery> function (type: string) { // get the model of type as mentioned in the argument } model.getCustomerDetails = part () { // get the details of customer who has purchased this model return 'customer details' } model.price = 100000 model.trackDelivery = role () { // track the delivery of the model return 'tracking address' } render model } let tesla = manufactureCar('tesla') tesla() // tesla is a function tesla.getCustomerDetails() // getCustomerDetails is a belongings divers on function
The object of type CarDelivery
is returned from the manufactureCar
function. The interface CarDelivery
helps in maintaining the shape of the object returned from the manufactureCar
part. Information technology makes sure that all the mandatory properties of the model —getCustomerDetails
, price
, and trackDelivery
— are present in the model.
How to utilise generics in interfaces
Generics in TypeScript are used when nosotros take to create generic components that can work on multiple data types. For example, we don't want to restrict our function to accept only number
as the input parameter. Information technology should calibration equally per the employ-instance and take a range of types.
Let'southward write code for implementing a stack that handles generic data types:
interface StackSpec<T> { (elements: Array<T>): void } part Stack<T> (elements) { this.elements = elements this.head = elements.length - 1 this.push = function (number): void { this.elements[this.head] = number this.head++ } this.pop = function <T>(): T { this.elements.splice(-one, i) this.head-- render this.elements[this.caput] } this.getElements = function (): Array<T> { return this.elements } } permit stacksOfStr: StackSpec<cord> = Stack let cars = new stacksOfStr(['Hatchback', 'Sedan', 'Land Rover']) cars.button('Tesla Model S') console.log('Cars', cars.getElements()) // ['Hatchback', 'Sedan', 'Land Rover', 'Tesla Model S']
The interface StackSpec
takes in any data-blazon and puts it in the definition of the part. T
is used for defining blazon
. The function Stack
takes an array of elements as the input. The Stack has methods —push
for adding a new element of blazon T
in the original elements
assortment, pop
is used for removing the meridian-almost element of the elements
assortment and getElements
function returns all the elements of type T
.
We've created a Stack of strings called stacksOfStr
, which takes in string
and appropriately replaces T
with cord
. We can reuse this stack implementation for creating stacks of number
and other data-types.
We can too create a stack of Tesla Models. Allow'due south run into how we can practice that:
let stacksOfTesla: StackSpec<TeslaModelS> = Stack allow teslaModels = [ { engineer: 'John', modelId: i, length: 112, //... }, // ... ] permit teslaStack = new stacksOfTesla(teslaModels) console.log(teslaStack) // prints the value of `teslaModels`
Please notation that we are using the same stack implementation for an array of type TeslaModelS
. Generics coupled with interfaces is a powerful tool in TypeScript.
How TypeScript compiles interfaces
TypeScript does a groovy chore in treatment the weird parts of JavaScript. However, the browser doesn't sympathise TypeScript and so it has to be compiled downwards to JavaScript.
The TypeScript compiler compiles the above TeslaModelSPrototype
grade equally:
var TeslaModelSPrototype = /** @class */ (function () { function TeslaModelSPrototype(fifty, westward, wb, sc) { this.length = l; this.width = w; this.wheelbase = wb; this.seatingCapacity = sc; } TeslaModelSPrototype.paradigm.getTyrePressure = function () { var tyrePressure = twenty; // Evaluated after doing a few complex computations! return tyrePressure; }; TeslaModelSPrototype.prototype.getRemCharging = function () { var remCharging = 20; // Evaluated afterwards doing a few complex computations! return remCharging; }; return TeslaModelSPrototype; }()); var teslaObj = new TeslaModelSPrototype(196, 86, 116, iv); console.log('Tyre Pressure level', teslaObj.getTyrePressure());
I'm using TypeScript Playground to see the compiled code. The instance variables —length
, width
, wheelBase
, and seatingCapacity
— are initialized in the function TeslaModelSPrototype
. The methods getTyrePressure
and getRemCharging
are defined on the prototype of the function TeslaModelSPrototype
.
The above code is plain JavaScript, so it can run in the browser.
Why utilise interfaces?
As you have already learned that the interfaces help in defining a concrete plan for the implementation of an entity. Autonomously from that, the interfaces also help in the performance of JavaScript engines. This section assumes that you've some agreement of JavaScript engines. In this section, nosotros'll dig deeper into the working of JavaScript engines and understand how interfaces help with the performance.
Let'south empathize how the Compiler sitting on V8 (JavaScript engine on Chrome) stores objects.
The interfaces in TypeScript be only until compile-time. As you lot can see in the to a higher place lawmaking that was generated by the TypeScript compiler, at that place is no mention of interfaces. The backdrop of TeslaModelS
interface (length
, width
, wheelBase
, and seatingCapacity
) are added in the TeslaModelSPrototype
constructor while the function types are attached on the epitome of TeslaModelSPrototype
function. The JavaScript engines don't know anything related to interfaces.
If nosotros instantiate thousands of TeslaModelSPrototype
cars, we will have to deal with thousands of objects of type TeslaModelS
. Each of these objects will have a structure similar to that of the interface. How does JavaScript engine store these thousands of objects of the same shape? Does it make thousands of copies of these objects? Making thousands of copies of similar shape is definitely a waste of retention. The JavaScript engines brand just one shape of type TeslaModelS
and each of the objects just stores corresponding values of the properties as defined in TeslaModelS
interface.
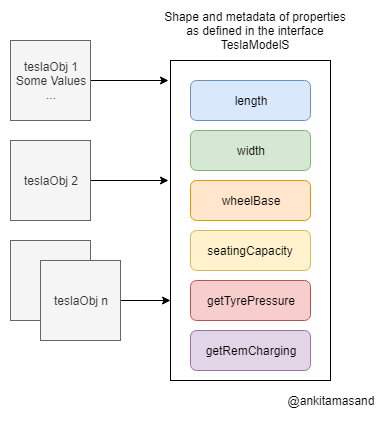
This is a great operation benefit on the side of JavaScript engines.
If the objects accept different shapes, the engines volition accept to create different shapes for these objects and handle them accordingly. Interfaces help in keeping the shapes of like objects intact.
How to utilize interfaces with React
Let's build a simple use-case of displaying the list of Pokemon using React and TypeScript interfaces.
Here's the main App Component that renders the Pokemon list in the div container with id root
:
import React, { Component, Fragment } from 'react'; import { render } from 'react-dom'; import PokemonList from './pokemon-list'; import './mode.css'; const App = () => { return ( <Fragment> <h2>Pokemon List</h2> <PokemonList /> </Fragment> ) } render(<App />, document.getElementById('root'));
The App
component renders PokemonList
.
Let'southward check the implementation of PokemonList
component:
import React, { Component } from 'react'; import { PokemonListModel } from './pokemon-model'; interface PokemonProps {} interface PokemonState { pokemonList: PokemonListModel | cipher; } form PokemonList extends Component<PokemonProps, PokemonState> { constructor (props) { super(props); this.state = { pokemonList: null } } getPokemonList = () => { fetch ('https://pokeapi.co/api/v2/pokemon/?limit=50') .and then (response => { return response.json(); }) .and then (response => { this.setState({ pokemonList: response }); }) } render () { let { pokemonList } = this.state; return ( <div className='pokemon-listing'> { pokemonList && pokemonList.results.map (pokemon => { render ( <div className='row' key={pokemon.proper noun}> <span>{pokemon.name}</bridge> </div> ) }) } </div> ) } componentDidMount () { this.getPokemonList() } } export default PokemonList
The PokemonList
component fetches the list of Pokemon using the open-source Poke API project. It stores the results of the Pokemon API in the land of the component. The component uses interfaces PokemonProps
and PokemonState
for defining its props
and state
. The interface PokemonListModel
defines the construction of an object as returned from the Pokemon API.
Here's the PokemonListModel
interface:
export interface PokemonListModel { count: number; side by side: cord | zippo; previous: string | null; results: Array } interface Pokemon { name: string; url: cord; }
Discover the type of results
property. It uses the interface Pokemon
to define the structure of results
. Hither's the demo of the Pokemon application on Stackblitz.
react-ts-pokemon – StackBlitz
List of Pokemon
Conclusion
Interfaces are a powerful way of defining contracts in TypeScript. Let'southward recap all that we take learned in this tutorial:
- Interfaces ascertain the specifications of entities and they tin exist implemented past functions or classes. We can define optional properties on an interface using
?
and read-but properties by using thereadonly
keyword in the property name - The TypeScript compiler also checks for backlog properties on an object and gives an error if an object contains a belongings that is divers in the interface
- We also learned how to define Indexable backdrop using interfaces
- Classes can implement an interface. The interface contains the definition for simply the case variables of a class
- Interfaces can exist extended to import properties of other interfaces using the
extends
keyword - We tin use the power of generics with interfaces and build reusable components
- Nosotros likewise learned how interfaces aid with the functioning of JavaScript engines
Full visibility into production React apps
Debugging React applications tin exist hard, especially when users experience issues that are hard to reproduce. If you're interested in monitoring and tracking Redux country, automatically surfacing JavaScript errors, and tracking deadening network requests and component load fourth dimension, try LogRocket.
LogRocket is like a DVR for web and mobile apps, recording literally everything that happens on your React app. Instead of guessing why problems happen, you can aggregate and report on what land your awarding was in when an issue occurred. LogRocket also monitors your app's performance, reporting with metrics like client CPU load, customer memory usage, and more.
The LogRocket Redux middleware package adds an extra layer of visibility into your user sessions. LogRocket logs all actions and state from your Redux stores.
Modernize how you debug your React apps — showtime monitoring for free.
Writing a lot of TypeScript? Watch the recording of our recent TypeScript meetup to learn most writing more than readable lawmaking.
TypeScript brings type condom to JavaScript. There tin can be a tension between type safety and readable code. Watch the recording for a deep swoop on some new features of TypeScript iv.iv.
Source: https://blog.logrocket.com/interfaces-in-typescript-what-are-they-and-how-do-we-use-them-befbc69b38b3/
0 Response to "How Do You Know Dups Values in the List Interface"
Post a Comment